In this article, we’ll go through what for loop in Java is and how you can take full advantage of it.
1. What is a For Loop in Java
Java for loop is a statement that allows you to run a set of commands, a predefined number of times(although if you use break
or continue
, you can reduce this number). The classic for loop is comprised of 4 parts all of which are optional:
- The initialization part
- The condition part
- The update part
- The body
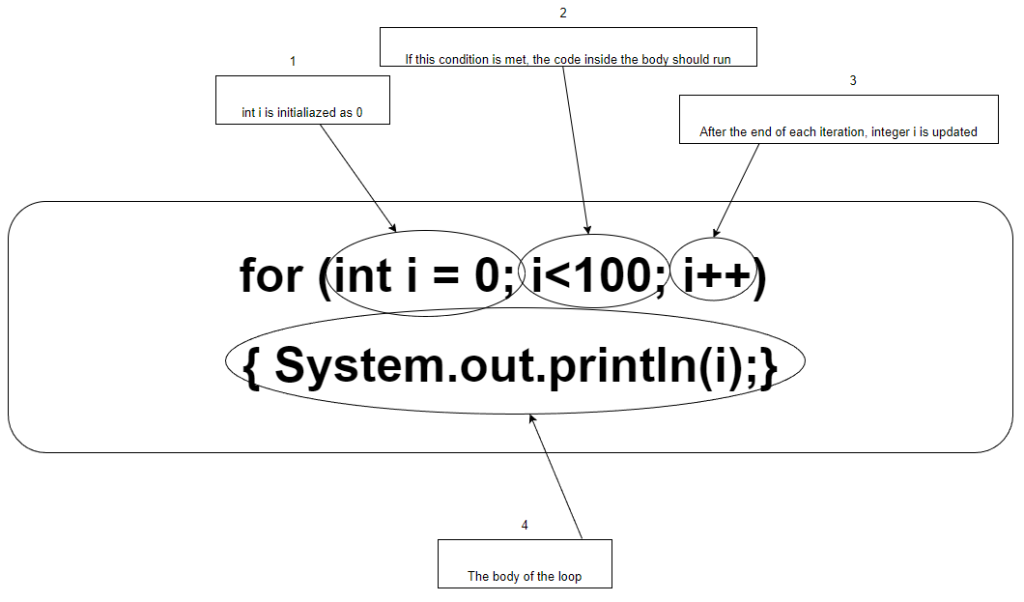
The above example will print numbers 0 to 99.
2. Java For Loop Syntax
Now that you have a basic understanding of what a for loop is, let’s see the syntax of for loop:
//When for loop has only one line of code, you can omit {} for (initialization statement(s); a boolean expression; update statement(s)) line_of_code; //For more than one lines for (initialization statement(s); a boolean expression; update statement(s)) { lines_of_code; }
2.1 Initialization Part
The initialization statement can be used to initialize more than one variable, as long as they have the same type, this means that you can have the following:
for( int i = 0, j = 100, k = 200; i == 100 || j == 200 || k == 200; i++, j++, k-- )
But NOT the following:
for(int i = 0, float k = 5; k < 20; i++,k++)
Moreover, the initialization part can be completely omitted as shown below:
int i = 0; for(; i < 100; i++)
2.2 The Condition Part
This is the most simple part of the for loop, as it should just be a boolean expression, or in other words, something that can be interpreted as either true or false.
So we could have any of the following:
// Run as long as i is less than 100 for(int i = 0; i < 100; i++) //Print the character as long as it is not 'L' String ylc = "You Learn Code"; for(int i = 0; ylc.charAt(i) != 'L'; i++ ) { System.out.println(ylc.charAt(i)); }
Note that this part is also optional, but if you do not add a condition that will end the loop, you may have created an infinite for loop as shown below:
//this will run forever for (;;) System.out.println("This will never end");
2.3 The Update Part
This part is used to update any value that was in the initialization part or before the loop. Also, this command is run after all commands inside the body of the loop have been executed Unlike the initialization part, you can update variables of different types; let’s see an example:
String ylc = "You Learn Code"; byte b = 1; for(int i = 0; i < 5; ylc += "-", b += 2, i++) System.out.println(ylc + " " + i + " " + b);
The snippet above will print the following:
You Learn Code 0 1 You Learn Code- 1 3 You Learn Code-- 2 5 You Learn Code--- 3 7 You Learn Code---- 4 9
As you can observe, we update not only the integer inside the initialization part but also the byte and the string variable.
2.4 The Body Part
The body part is simply the code that will run, every time the condition evaluates to true. If the condition never evaluates to true, the code inside the {} will never run.
3. Nested For Loop in Java
For loops can also be nested, exactly like if-else statements. Let’s see an example:
for (int i = 0; i < 3; i++) { for (int j = 3; j >= 0; j--){ System.out.println("i is:"+ i + " j is:" + j); } }
The most usual case for nested loops is traversing a 2-d array or an array with more dimensions. Moreover, you can label a for loop in order to be able to identify it when you use the break or continue keyword, but first, let’s see briefly what break and continue are:
- The
break
keyword is an instruction to exit the loop - The
continue
keyword is an instruction to skip the current iteration and proceed with the next one
Now, let’s take the previous example and modify it a little:
for (int i = 0; i < 3; i++) { for (int j = 3; j >= 0; j--){ if(j == 1) break; if(j == 2) continue; System.out.println("i is:"+ i + " j is:" + j); } }
The above example will result in exiting the inner loop(the one with the j variable) when j is equal to 1, while if j is equal to 2, the loop will continue to the next iteration(in this example, the next iteration will be j = 1).
Now, what if we would like to completely break out of all loops when a condition is met? That’s why we need the labeled loops:
outer: for (int i = 0; i < 3; i++) { inner: for (int j = 3; j >= 0; j--){ if(j == 1) break outer; if(j == 2) continue; System.out.println("i is:"+ i + " j is:" + j); } }
With the use of labels, we can define which loop we want to exit from, and in this case, we chose to exit from the outer loop. Likewise, you can use labeled loops with the continue keyword.
4. Enhanced For Loop
The enhanced for loop is a specific type of loop with a different syntax:
for (Object_type_or_primitive local_var_name : a_collection_or_array_name) { //code }
Below, you can find two examples of enhanced for loop in action:
int[] numbers = new int[]{1, 2, 3, 4, 5}; for (int i : numbers) { System.out.println(i); } // Will print 1, 2, 3, 4, 5 List<String> ylc = List.of("You", "Learn", "Code"); for (String s: ylc) { System.out.println(s); } //Will print You Learn Code
One big difference with the classic for loop is that this loop is read-only, as we do not have the index as in the classic loop.
If you want to dig deep into the enhanced for loop, you can check For Each Loop in Java article.
5. For Loop Example – Find if a Word is a Palindrome
You can find if a word is a palindrome with just a for loop and a couple lines of code:
boolean isPalindrome(String word) { for ( int i = 0, j = word.length() - 1; i <= word.length() / 2 || j >= word.length()/2; i++, j-- ){ if(word.charAt(i) != word.charAt(j)) return false; } return true; }
Let’s explain how this works:
- We have two pointers, i, which points to the index 0 and increments, and j, which points to the index of the last character and it decrements
- We do not have to go through all the characters twice as at some point the two pointers will meet
- If we find a character that does not match, this means that this word is not a palindrome so we return false
Now let’s run it with multiple words:
isPalindrome("anna"); // true isPalindrome("kayak"); // true isPalindrome("deified"); // true isPalindrome("peep"); // true isPalindrome("level"); // true isPalindrome("hello"); // false isPalindrome("world"); // false isPalindrome("you"); // false
6. Conclusion
By now, you should know how to use a for loop in java. You can find the source code on our GitHub page.
Leave a Reply